Part - II | Part I | Part III | Part IV
Project
- Server
- Deployment
Requirements :
1.Project Preparation
Code Review
- Conduct a thorough review of your Django code for any potential issues, bugs, and areas for improvement.
- Ensure code quality, readability, and maintainability.
Testing
- Run comprehensive tests, including unit tests, integration tests, and end-to-end tests.
- Verify that all functionalities are working as expected.
Static File Collection
- Use python manage.py collectstatic to gather all static files (CSS, JavaScript, images) into a single directory.
- This simplifies deployment and improves performance.
Virtual Environment
- Create a virtual environment to isolate project dependencies from the system-wide Python installation.
- This prevents conflicts and ensures consistent behavior across environments.
Requirements File
- Create a requirements.txt file to list all project dependencies.
- This file makes it easy to install all necessary packages on the server during deployment.
Django Project Structure
Django Project
├── <projectname>/
│ ├── __init__.py
│ ├── settings.py
│ ├── urls.py
│ ├── wsgi.py
└── manage.py
__init__.py : An empty __init__.py file in a directory tells Python that the directory is a package. A package is a way to organize Python code into reusable modules. When you import a package, the __init__.py file is executed (if it exists). You can also add initialization code to this file, such as importing submodules, defining variables, or executing other code.
settings.py : In essence, the settings.py file acts as the command center for your Django project, dictating how various components interact and behave. You can customize these settings to tailor your Django application to your specific needs.The settings.py file is a crucial configuration module in Django applications. It contains settings and configurations for the Django project, such as database configurations, static files management, middleware settings, installed apps, and security settings. This file allows developers to customize the behavior of their Django application according to the project's requirements, managing elements like allowed hosts, debug mode, and internationalization settings. Adjusting parameters in settings.py lets developers tailor the framework to suit their environment, whether in development or production.
urls.py : The urls.py file in a Django application is responsible for routing URLs to the appropriate views. It acts as a mapping between URL patterns and view functions, allowing the framework to determine which view to execute based on the incoming request's URL. Each URL pattern can include dynamic segments, enabling developers to create clean and user-friendly URLs. By organizing and defining these routes in urls.py, developers can control navigation within the application and facilitate the handling of web requests effectively.
wsgi.py : WSGI, or Web Server Gateway Interface, is a specification that facilitates communication between web servers and Python web applications. It serves as a standard interface, allowing web servers like Apache or NGINX to forward requests to Python applications, such as those built with frameworks like Django or Flask, and subsequently relay responses back to the client.
Popular WSGI Servers to Know
Gunicorn (Green Unicorn)
Gunicorn is a pre-fork worker model-based server that’s compatible with various web frameworks. Additionally, it’s simple to implement.
uWSGI
uWSGI is known for being a highly-performant WSGI server implementation. uWSGI can be used for the development and deployment of a Python application. In uWSGI, application servers, proxies, process managers and monitors are all implemented through a common API and a common configuration style. This makes it a developer-friendly WSGI server.
mod_wsgi
mod_wsgi is a Python package that provides an Apache module that implements a WSGI compliant interface for hosting Python-based web applications on top of the Apache web server.
CherryPy
CherryPy is a Python web framework that allows developers to create web applications in the same way by which they develop other object-oriented Python programs. CherryPy is considered to be a reliant object-oriented HTTP framework that can efficiently run on multiple HTTP servers at once.
manage.py : In summary, manage.py is an essential tool for Django developers, streamlining project management, database operations, application handling, and testing processes.Project Management: It provides a straightforward way to manage and interact with your Django project, eliminating the need for complex command-line arguments by offering a set of predefined commands12.
Development Server: With manage.py, developers can easily start a local development server to test and debug their applications before deploying them to production12.
Database Operations: The file facilitates database management tasks such as creating tables, applying migrations, and synchronizing the database schema with Django models12.
Application Management: It allows for the creation of new Django applications within the project and handles tasks related to static files and application configurations12.
Testing and Debugging: manage.py includes commands for running tests and checking the overall health of the application, aiding in efficient issue identification and resolution
Server:
Create Instance (EC2)
EC2 stands for Elastic Compute Cloud. EC2 is an on-demand computing service on the AWS cloud platform. Under computing, it includes all the services a computing device can offer to you along with the flexibility of a virtual environment. It also allows the user to configure their instances as per their requirements, i.e., allocate the RAM, ROM, and storage according to the need of the current task. Even the user can dismantle the virtual device once its task is completed and it is no more required. For providing all these scalable resources, AWS charges some bill amount at the end of every month; the bill amount is entirely dependent on your usage. EC2 allows you to rent virtual computers. The provision of servers on AWS Cloud is one of the easiest ways in EC2. EC2 has resizable capacity. EC2 offers security, reliability, high performance, and cost-effective infrastructure so as to meet the demanding business needs.
- Choose Hosting Provider (Amazon Web Service.Google Cloud,Microsoft Azure)
- Server OS (Amazon Linux,Ubuntu,Suse,Windows)
- Install Python and Dependencies (Django,Pillow,psycopg2-binary,numpy ...)
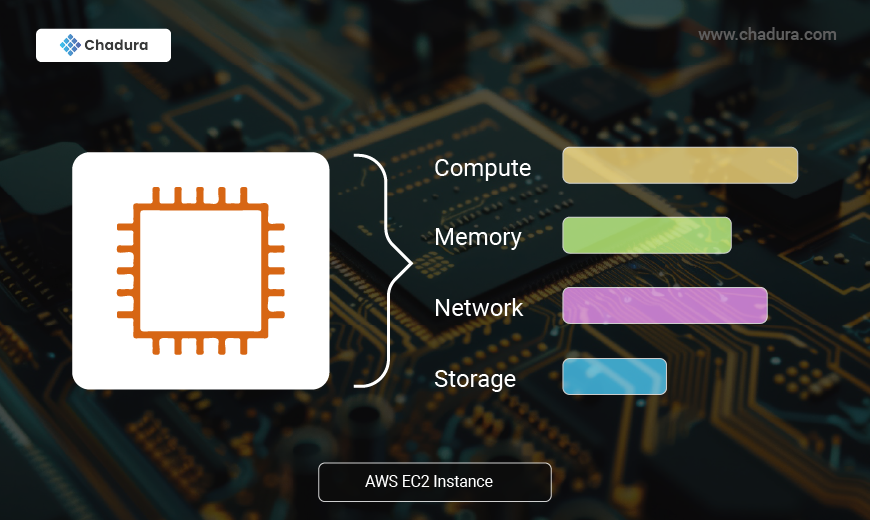
Creating Instance Ec2 : Sign in to the AWS Management Console --> Click on the EC2 service --> Click on the Launch Instance button to create a new instance - For more Click here
Deployment
Manual: Manually copy files to the server using tools like scp or rsync.
Automated: Use tools like Fabric, Ansible, or CI/CD pipelines (Jenkins, GitLab CI) for Security:
Real-Time Deployment Techniques
Requirement Files
1.gunicorn.sh
2.requirements.txt
3. . env
4.Restart_Server.sh
1.gunicorn.sh
Gunicorn is a robust choice for deploying Python web applications due to its performance and ease of use. By leveraging systemd service scripts, developers can efficiently manage their applications in production environments.
It include some commands like
#!/bin/bash
NAME="projectname" # Name of the application
DJANGODIR=/home/debian/myproject # Django project directory
SOCKFILE=/home/debian/myproject/env/run/gunicorn.sock # we will communicte using this unix socket
USER=debian # the user to run as
GROUP=debian # the group to run as
NUM_WORKERS=3 # how many worker processes should Gunicorn spawn
DJANGO_SETTINGS_MODULE=src.projectname.settings # which settings file should Django use
DJANGO_WSGI_MODULE=src.projectname.wsgi # WSGI module name
echo "Starting $NAME as `whoami`"
# Activate the virtual environment
cd $DJANGODIR
source /home/debian/myproject/env/bin/activate
export DJANGO_SETTINGS_MODULE=$DJANGO_SETTINGS_MODULE
export PYTHONPATH=$DJANGODIR:$PYTHONPATH
# Create the run directory if it doesn't exist
RUNDIR=$(dirname $SOCKFILE)
test -d $RUNDIR || mkdir -p $RUNDIR
# Start your Django Unicorn
# Programs meant to be run under supervisor should not daemonize themselves (do not use --daemon)
exec gunicorn ${DJANGO_WSGI_MODULE}:application \
--name $NAME \
--workers $NUM_WORKERS \
--user=$USER --group=$GROUP \
--bind=unix:$SOCKFILE \
--log-level=debug \
--log-file=-
2.requirements.txt
requirements.txt file is essential for managing dependencies in Python projects, facilitating easier installations and ensuring that applications run as intended across various setups.developers can manually create the file by listing each dependency on a new line, formatted as package_name==version
3. .env
. env files are essential for managing environment-specific configurations securely and efficiently, making them a best practice in modern software development.
This is include:
# Database Configuration
DB_HOST=localhost
DB_USER=root
DB_PASS=password# API Keys
API_KEY=your_api_key_here
Libraries like dotenv in Node.js or python-dotenv in Python are commonly used to read these .env files and load the variables into the application’s environment at runtime. This allows the application to access these variables as if they were set in the operating system's environment.
4.Restart_Server.sh:
This command temporarily sets SELinux to permissive mode.The change is temporary and will revert to the previous state (enforcing mode) upon the next system reboot. To make a permanent change, you would need to edit the /etc/selinux/config file and set SELINUX=permissive or SELINUX=disabled
sudo setenforce 0
This command stops the Nginx service and then starts it again. It is typically used after making significant changes to the Nginx configuration files, such as modifying server blocks or changing ports.
sudo systemctl restart nginx
It is used to stop a process named "projectname" that is being managed by Supervisor, a process control system.This command instructs Supervisor to terminate the "acharya" process gracefully.
sudo supervisorctl stop projectname
It is used to start a process named "projectname" that is managed by Supervisor, a process control system.
sudo supervisorctl start projectname
It is used to restart the Supervisor daemon and apply any configuration changes made to the processes it manages.
sudo supervisorctl reload
Comments (0)